04/01/2024
16 min read
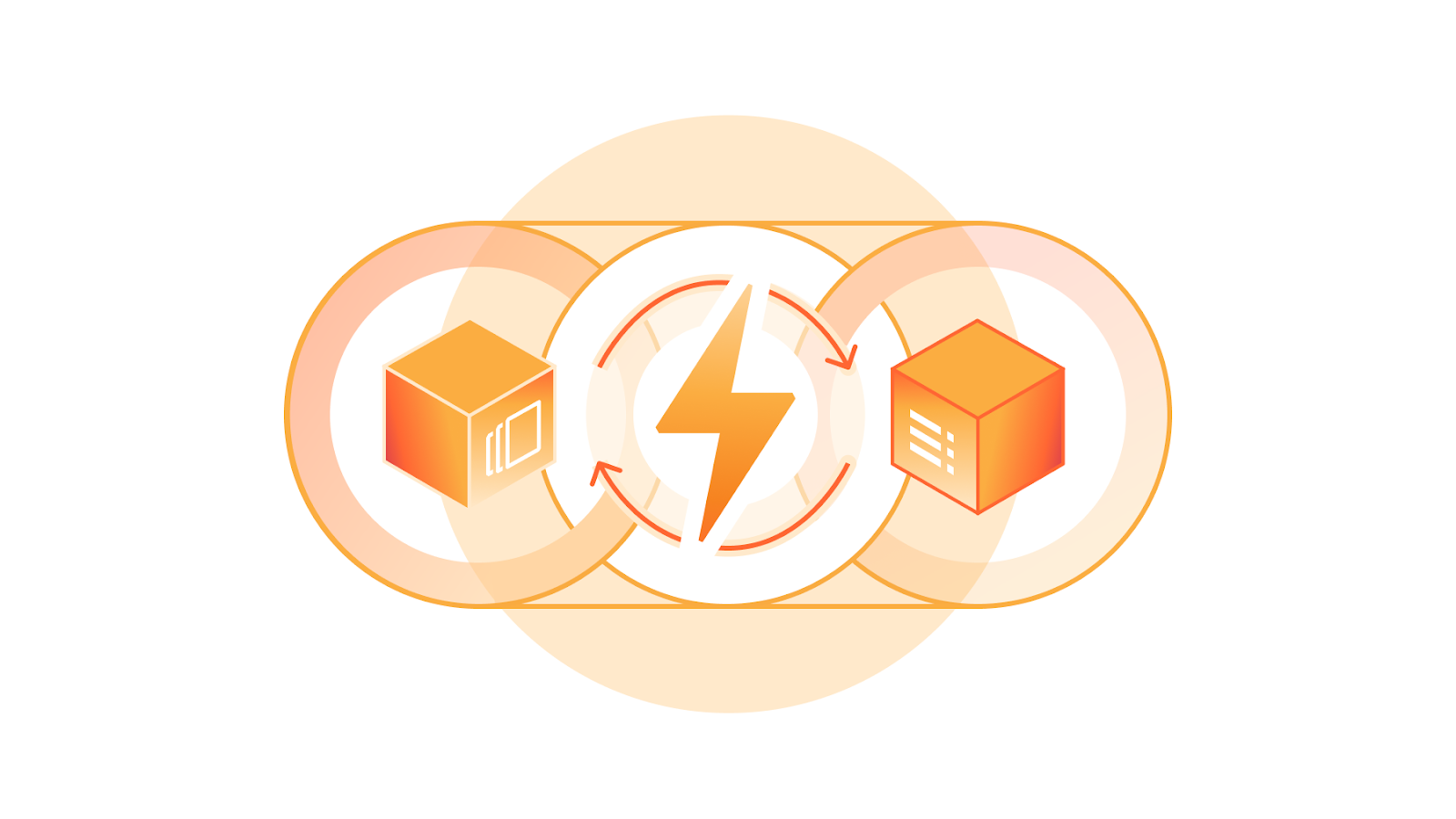
If you've ever written a Cloudflare Worker using Workers KV for storage, you may have noticed something unsettling.
// A simple Worker that always returns the value named "content",
// read from Workers KV storage.
export default {
async fetch(request, env, ctx) {
return new Response(await env.MY_KV.get("content"));
}
}
Do you feel something is… missing? Like… Where is the setup? The authorization keys? The client library instantiation? Aren't environment variables normally strings? How is it that env.MY_KV
seems to be an object with a get()
method that is already hooked up?
Coming from any other platform, you might expect to see something like this instead:
// How would a "typical cloud platform" do it?
// Import KV client library?
import { KV } from "cloudflare:kv";
export default {
async fetch(request, env, ctx) {
// Connect to the database?? Using my secret auth key???
// Which comes from an environment variable????
let myKv = KV.connect("my-kv-namespace", env.MY_KV_AUTHKEY);
return new Response(await myKv.get("content"));
}
}
As another example, consider service bindings, which allow a Worker to send requests to another Worker.
// A simple Worker that greets an authenticated user, delegating to a
// separate service to perform authentication.
export default {
async fetch(request, env, ctx) {
// Forward headers to auth service to get user info.
let authResponse = await env.AUTH_SERVICE.fetch(
"https://auth/getUser",
{headers: request.headers});
let userInfo = await authResponse.json();
return new Response("Hello, " + userInfo.name);
}
}
Notice in particular the use of env.AUTH_SERVICE.fetch()
to send the request. This sends the request directly to the auth service, regardless of the hostname we give in the URL.
On "typical” platforms, you'd expect to use a real (perhaps internal) hostname to route the request instead, and also include some credentials proving that you're allowed to use the auth service API:
// How would a "typical cloud platform" do it?
export default {
async fetch(request, env, ctx) {
// Forward headers to auth service, via some internal hostname?
// Hostname needs to be configurable, so get it from an env var.
let authRequest = new Request(
"https://" + env.AUTH_SERVICE_HOST + "/getUser",
{headers: request.headers});
// We also need to prove that our service is allowed to talk to
// the auth service API. Add a header for that, containing a
// secret token from our environment.
authRequest.headers.set("X-Auth-Service-Api-Key",
env.AUTH_SERVICE_API_KEY);
// Now we can make the request.
let authResponse = await fetch(authRequest);
let userInfo = await authResponse.json();
return new Response("Hello, " + userInfo.name);
}
}
As you can see, in Workers, the "environment" is not just a bunch of strings. It contains full-fledged objects. We call each of these objects a "binding", because it binds the environment variable name to a resource. You configure exactly what resource a name is bound to when you deploy your Worker – again, just like a traditional environment variable, but not limited to strings.
We can clearly see above that bindings eliminate a little bit of boilerplate, which is nice. But, there's so much more.
Bindings don't just reduce boilerplate. They are a core design feature of the Workers platform which simultaneously improve developer experience and application security in several ways. Usually these two goals are in opposition to each other, but bindings elegantly solve for both at the same time.
Security
It may not be obvious at first glance, but bindings neatly solve a number of common security problems in distributed systems.
SSRF is Not A Thing
Bindings, when used properly, make Workers immune to Server-Side Request Forgery (SSRF) attacks, one of the most common yet deadly security vulnerabilities in application servers today. In an SSRF attack, an attacker tricks a server into making requests to other internal services that only it can see, thus giving the attacker access to those internal services.
As an example, imagine we have built a social media application where users are able to set their avatar image. Imagine that, as a convenience, instead of uploading an image from their local disk, a user can instead specify the URL of an image on a third-party server, and the application server will fetch that image to use as the avatar. Sounds reasonable, right? We can imagine the app contains some code like:
let resp = await fetch(userAvatarUrl);
let data = await resp.arrayBuffer();
await setUserAvatar(data);
One problem: What if the user claims their avatar URL is something like "https://auth-service.internal/status"? Whoops, now the above code will actually fetch a status page from the internal auth service, and set it as the user's avatar. Presumably, the user can then download their own avatar, and it'll contain the content of this status page, which they were not supposed to be able to access!
But using bindings, this is impossible: There is no URL that the attacker can specify to reach the auth service. The application must explicitly use the binding env.AUTH_SERVICE
to reach it. The global fetch()
function cannot reach the auth service no matter what URL it is given; it can only make requests to the public Internet.
A legacy caveat: When we originally designed Workers in 2017, the primary use case was implementing a middleware layer in front of an origin server, integrated with Cloudflare's CDN. At the time, bindings weren't a thing yet, and we were primarily trying to implement the Service Workers interface. To that end, we made a design decision: when a Worker runs on Cloudflare in front of some origin server, if you invoke the global fetch()
function with a URL that is within your zone's domain, the request will be sent directly to the origin server, bypassing most logic Cloudflare would normally apply to a request received from the Internet. Sadly, this means that Workers which run in front of an origin server are not immune to SSRF – they must worry about it just like traditional servers on private networks must. Although this puts Workers in the same place as most servers, we now see a path to make SSRF a thing you never have to worry about when writing Workers. We will be introducing "origin bindings", where the origin server is represented by an explicit binding. That is, to send a request to your origin, you'd need to do env.ORIGIN.fetch()
. Then, the global fetch()
function can be restricted to only talk to the public Internet, fully avoiding SSRF. This is a big change and we need to handle backwards-compatibility carefully – expect to see more in the coming months. Meanwhile, for Workers that do not have an origin server behind them, or where the origin server does not rely on Cloudflare for security, global fetch()
is SSRF-safe today.
And a reminder: Requests originating from Workers have a header, CF-Worker
, identifying the domain name that owns the Worker. This header is intended for abuse mitigation: if your server is receiving abusive requests from a Worker, it tells you who to blame and gives you a way to filter those requests. This header is not intended for authorization. You should not implement a private API that grants access to your Workers based solely on the CF-Worker
header matching your domain. If you do, you may re-open the opportunity for SSRF vulnerabilities within any Worker running on that domain.
You can't leak your API key if there is no API key
Usually, if your web app needs access to a protected resource, you will have to obtain some sort of an API key that grants access to the resource. But typically anyone who has this key can access the resource as if they were the Worker. This makes handling auth keys tricky. You can't put it directly in a config file, unless the entire config file is considered a secret. You can't check it into source control – you don't want to publish your keys to GitHub! You probably shouldn't even store the key on your hard drive – what if your laptop is compromised? And so on.
Even if you have systems in place to deliver auth keys to services securely (like Workers Secrets), if the key is just a string, the service itself can easily leak it. For instance, a developer might carelessly insert a log statement for debugging which logs the service's configuration – including keys. Now anyone who can access your logs can discover the secret, and there's probably no practical way to tell if such a leak has occurred.
With Workers bindings, we endeavor for bindings to be live objects, not secret keys. For instance, as seen in the first example in this post, when using a Workers KV binding, you never see a key at all. It's therefore impossible for a Worker to accidentally leak access to a KV namespace.
No certificate management
This is similar to the API key problem, but arguably worse. When internal services talk to each other over a network, you presumably want them to use secure transports, but typically that requires that every service have a certificate and a private key signed by some CA, and clients must be configured to trust that CA. This is all a big pain to manage, and often the result is that developers don't bother; they set up a VPC and assume the network is trusted.
In Workers, since all intra-service communications happen over a binding, the system itself can take on all the work of ensuring the transport is secure and goes to the right place.
No frustrating ACL management – but also no lazy "allow all"
At this point you might be thinking: Why are we talking about API keys at all? Cloudflare knows which Worker is sending any request. Can't it handle the authentication that way?
Consider the earlier example where we imagined that KV namespaces could be opened by name:
// Imagine KV namespaces could be open by name?
let myKv = KV.connect("my-kv-namespace", env.MY_KV_AUTHKEY);
What if we made it simply:
// No authkey, because the system knows whether the Worker has
// permission?
let myKv = KV.connect("my-kv-namespace");
We could then imagine that we could separately configure each KV namespace with an Access Control List (ACL) that specifies which Workers can access it.
Of course, the first problem with this is that it's vulnerable to SSRF. But, we discussed that already, so let's discuss another problem.
Many platforms use ACLs for security, but have you ever noticed how everyone hates them? You end up with two choices:
- Tediously maintain ACLs on every resource. Inevitably, this is always a huge pain. First you deploy your code, which you think is properly configured. Then you discover that it's failing with permissions errors causing a production outage! So you go fiddle with the IAM system. There are 533,291 roles to choose from and none of them are actually what you want. It turns out you're supposed to create a custom role, but that's not obvious, and once you get there, the UI is confusing. Also it's easy to confuse your team's service account with your team's email group, so you give the permissions to the wrong principal, but it takes you an hour of staring at it to realize what you did wrong. Then somehow you manage to remove your own access to the resource and you can't add it back even though you're a project admin? (Why yes, all this did in fact happen to me, while using a cloud provider that shall remain nameless.)
- Give up and grant everything access to everything. Just put all your services in a single VPC where they can all freely talk to each other. This is what most developers are inclined to do, if their security team doesn't step in to stop them.
Much of this pain comes about because connecting a server to a resource today involves two steps that should really be one step:
- Configure the server to point at the resource.
- Configure the resource to accept requests from the server.
Developers are primarily concerned with step 1, and forget that step 2 exists until it blows up in their faces. Then it's a mad scramble to learn how step 2 even works.
What if step 1 just implied step 2? Obviously, if you're trying to configure a service to access a resource, then you also want the resource to allow access to the service. As long as the person trying to set this up has permissions to both, then there is no reason for this to be a two-step process.
But in typical platforms, the platform itself has no way of knowing that a service has been configured to talk to a resource, because the configuration is just a string.
Bindings fix that. When you define a binding from a Worker to a particular KV namespace, the platform inherently understands that you are telling the Worker to use the KV namespace. Therefore, it can implicitly ensure that the correct permissions are granted. There is no step 2.
And conversely, if no binding is configured, then the Worker does not have access. That means that every Worker starts out with no access by default, and only receives access to exactly the things it needs. Secure by default.
As a related benefit, you can always accurately answer the question "What services are using this resource?" based on bindings. Since the system itself understands bindings and what they point to, the system can answer the query without knowing anything about the service's internals.
Developer Experience
We've seen that bindings improve security in a number of ways. Usually, people expect security and developer friendliness to be a trade-off, with each security measure making life harder for developers. Bindings, however, are entirely the opposite! They actually make life easier!
Easier setup
As we saw in the intro, using a binding reduces setup boilerplate. Instead of receiving an environment variable containing an API key which must be passed into some sort of library, the environment variable itself is an already-initialized client library.
Observability
Because the system understands what bindings a Worker has, and even exactly when those bindings are exercised, the system can answer a lot of questions that would normally require more manual instrumentation or analysis to answer, such as:
- For a given Worker, what resources does it use? Since the system understands the types of all bindings and what they point to (it doesn't just see them as opaque strings), it can answer this question.
- For a given resource, which Workers use it? This is the reverse query. The system can maintain an index of bindings in order to find ones pointing at a given resource.
- How often does a particular Worker use a particular resource? Since bindings are invoked by calling methods on the binding itself, the system can observe these calls, log them, collect metrics, etc.
Testability via dependency injection
When you deploy a test version of your service, you probably want it to operate on test resources rather than real production resources. For instance, you might have a separate testing KV namespace for storage. But, you probably want to deploy exactly the same code to test that you will eventually deploy to production. That means the names of these resources cannot be hard-coded.
On traditional platforms, the obvious way to avoid hard-coding resource names is to put the name in an environment variable. Going back to our example from the intro, if KV worked in a traditional way not using bindings, you might end up with code like this:
// Hypothetical non-binding-based KV.
let myKv = KV.connect(env.MY_KV_NAMESPACE, env.MY_KV_AUTHKEY);
At best, you now have two environment variables (which had better stay in sync) just to specify what namespace to use.
But at worst, developers might forget to parameterize their resources this way.
- A developer may write new code that is hard-coded to use a test database, and then forget to update it before pushing it to production, accidentally using the test database in prod.
- A developer might prototype a new service using production resources from the start (or using new resources which become production resources), only later on deciding that they need to create a new deployment for testing. But by then, it may be a pain to find and parameterize all the different resources used.
With bindings, it's impossible to have this problem. Since you can only connect to a KV namespace through a binding, it's always possible to make a separate deployment of the same code which talks to a test namespace instead of production, e.g. using Wrangler Environments.
In the testing world, this is sometimes called "dependency injection". With bindings, dependencies are always injectable.
Adaptability
Dependency injection isn't just for tests. A service whose dependencies can be changed out easily will be easier to deploy into new environments, including new production environments.
Say, for instance, you have a service that authenticates users. Now you are launching a new product, which, for whatever reason, has a separate userbase from the original product. You need to deploy a new version of the auth service that uses a different database to implement a separate user set. As long as all dependencies are injectable, this should be easy.
Again, bindings are not the only way to achieve dependency injection, but a bindings-based system will tend to lead developers to write dependency-injectable code by default.
Q&A
Has anyone done this before?
You have. Every time you write code.
As it turns out, this approach is used all the time at the programming language level. Bindings are analogous to parameters of a function, or especially parameters to a class constructor. In a memory-safe programming language, you can't access an object unless someone has passed you a pointer or reference to that object. Objects in memory don't have URLs that you use to access them.
Programming languages work this way because they are designed to manage complexity, and this proves to be an elegant way to do so. Yet, this style which we're used to using at the programming language level is much less common at the distributed system level. The Cloudflare Workers platform aims to treat the network as one big computer, and so it makes sense to extend programming language concepts across the network.
Of course, we're not the first to apply this to distributed systems, either. The paradigm is commonly called "capability-based security", which brings us to the next question…
Is this capability-based security?
Bindings are very much inspired by capability-based security.
At present, bindings are not a complete capability system. In particular, there is currently no particular mechanism for a Worker to pass a binding to another Worker. However, this is something we can definitely imagine adding in the future.
Imagine, for instance, you want to call another Worker through a service binding, and as you do, you want to give that other Worker temporary access to a KV namespace for it to operate on. Wouldn't it be nice if you could just pass the object, and have it auto-revoked at the end of the request? In the future, we might introduce a notion of dynamic bindings which can bind to different resources on a per-request basis, where a calling Worker can pass in a particular value to use for a given request.
For the time being, bindings cannot really be called object capabilities. However, many of the benefits of bindings are the same benefits commonly attributed to capability systems. This is because of some basic similarities:
- Like a capability, a binding simultaneously designates a resource and also confers permission to access that resource, without referencing any separate ACL.
- Like capabilities, bindings do not exist in any global namespace: they are scoped to the env object passed to a specific Worker.
- Like a capability, to use a binding, the application must explicitly specify which binding it is trying to use, and only specifies the binding. In particular, the application does not separately specify the name of the resource in any other namespace (no URL, no global ID, etc.). The existence of the binding only affects the application's behavior when the application explicitly invokes that binding.
Why is env a parameter to fetch(), not global?
This is a bit wonky, but the goal is to enable composition of Workers.
Imagine you have two Workers, one which implements your API, mapped to api.example.com, and one which serves static assets, mapped to assets.example.com. One day, for whatever reason, you decide you want to combine these two Workers into a single Worker. So you write this code:
import apiWorker from "api-worker.js";
import assetWorker from "asset-worker.js";
export default {
async fetch(req, env, ctx) {
let url = new URL(req.url);
if (url.hostname == "api.example.com") {
return apiWorker.fetch(req, env, ctx);
} else if (url.hostname == "assets.example.com") {
return assetWorker.fetch(req, env, ctx);
} else {
return new Response("Not found", {status: 404});
}
}
}
This is great! No code from either Worker needed to be modified at all. We just create a new file containing a router Worker that delegates to one or the other.
But, you discover a problem: both the API Worker and the assets Worker use a KV namespace binding, and it turns out that they both decided to name the binding env.KV, but these bindings are meant to point to different namespaces used for different purposes. Does this mean I have to go edit the Workers to change the name of the binding before I can merge them?
No, it doesn't, because I can just remap the environments before delegating:
import apiWorker from "api-worker.js";
import assetWorker from "asset-worker.js";
export default {
async fetch(req, env, ctx) {
let url = new URL(req.url);
if (url.hostname == "api.example.com") {
let subenv = {KV: env.API_KV};
return apiWorker.fetch(req, subenv, ctx);
} else if (url.hostname == "assets.example.com") {
let subenv = {KV: env.ASSETS_KV};
return assetWorker.fetch(req, subenv, ctx);
} else {
return new Response("Not found", {status: 404});
}
}
}
If environments were globals, this remapping would not be possible.
In fact, this benefit goes much deeper than this somewhat-contrived example. The fact that the environment is not a global essentially forces code to be internally designed for dependency injection (DI). Designing code to be DI-friendly sometimes seems tedious, but every time I've done it, I've been incredibly happy that I did. Such code tends to be much easier to test and to adapt to new circumstances, for the same reasons mentioned when we discussed dependency injection earlier, but applying at the level of individual modules rather than whole Workers.
With that said, if you really insist that you don't care about making your code explicitly DI-friendly, there is an alternative: Put your env into AsyncLocalStorage. That way it is "ambiently" available anywhere in your code, but you can still get some composability.
import { AsyncLocalStorage } from 'node:async_hooks';
// Allocate a new AsyncLocalStorage to store the value of `env`.
const ambientEnv = new AsyncLocalStorage();
// We can now define a global function that reads a key from env.MY_KV,
// without having to pass `env` down to it.
function getFromKv(key) {
// Get the env from AsyncLocalStorage.
return ambientEnv.getStore().MY_KV.get(key);
}
export default {
async fetch(req, env, ctx) {
// Put the env into AsyncLocalStorage while we handle the request,
// so that calls to getFromKv() work.
return ambientEnv.run(env, async () => {
// Handle request, including calling functions that may call
// getFromKv().
// ... (code) ...
});
}
};
How does a KV binding actually work?
Under the hood, a Workers KV binding encapsulates a secret key used to access the corresponding KV namespace. This key is actually the encryption key for the namespace. The key is distributed to the edge along with the Worker's code and configuration, using encrypted storage to keep it safe.
Although the key is distributed with the Worker, the Worker itself has no way to access the key. In fact, even the owner of the Cloudflare account cannot see the key – it is simply never revealed outside of Cloudflare's systems. (Cloudflare employees are also prevented from viewing these keys.)
Even if an attacker somehow got ahold of the key, it would not be useful to them as-is. Cloudflare's API does not provide any way for a user to upload a raw key to use in a KV binding. The API instead has the client specify the public ID of the namespace they want to use. The deployment system verifies that the KV namespace in question is on the same account as the Worker being uploaded (and that the client is authorized to deploy Workers on said account).
Get Started
To learn about all the types of bindings offered by Workers and how to use them, check out the documentation.
We protect entire corporate networks, help customers build Internet-scale applications efficiently, accelerate any website or Internet application, ward off DDoS attacks, keep hackers at bay, and can help you on your journey to Zero Trust.
Visit 1.1.1.1 from any device to get started with our free app that makes your Internet faster and safer.
To learn more about our mission to help build a better Internet, start here. If you're looking for a new career direction, check out our open positions.